How to import arbitrary data into a jit.matrix?
I had hoped to populate a jit.matrix with algorithmically-generated data (perhaps from another environment).
I could find two ways to do this:
- Read a text file using Max objects and stick the data into the matrix, cell by cell, using "setcell" messages (like, 10000 of them for a 100-by-100 matrix).
- Or, write a JXF file (i.e. write my own functions to produce the binary format).
Is there an easier way?
hjh
uh... jit.fill
> uh... jit.fill
Let me then revise my question.
Is there an established methodology, or set of best practices, for using jit.fill to initialize a multiplane matrix?
For instance, in a scripting language such as SuperCollider, to initialize an array (one dimension), you write the values inside square brackets, separated by commas. To initialize a two-dimensional array, you do the same, except each value is itself an array in square brackets, and so on for more dimensions. This is easy to describe and easy to learn.
AFAICS the same in Jitter requires a series of messages to two different objects:
```
// to jit.matrix
planecount n
dim xsize ysize
// to jit.fill
plane 0
(list of data)
plane 1
(list of data)
... etc
```
In the specific use case, the data set won't be extremely large (mainly looking to drive a jit.gl.multiple), so this is manageable, but it's also just clumsy enough that I wonder if I might have overlooked an example somewhere of a better way?
hjh
You set the size of the matrix with messages (dim, planecount...).
Then you use jit.fill (if you are working with lists, otherwise messages such as setcell will work just fine as well) to fill the matrix proper.
Should be classic enough. Did you try it?
> Then you use jit.fill (if you are working with lists, otherwise messages such as setcell will work just fine as well) to fill the matrix proper.
But, as noted, if you have (for instance) a list of positions for jit.gl.multiple such as x0 y0 z0 x1 y1 z1 x2 y2 z2... (because I can imagine a lot of users thinking of their coordinates in these terms), then to use jit.fill, you need some logic to collect all of the x* values into one list, and all of the y* and z* values into separate lists, because jit.fill is oriented only toward planes. It has no option to fill by cells (leaving you with cell-by-cell "setcell" calls... OK, if that's what it comes down to).
So I'm wondering if this already exists somewhere, as an abstraction.
Here's my thinking... Jitter does get it right by making matrices arbitrary data (unlike, say, Gem in Pd, where you only have pix buffers and you can't use them for anything else). So I look at that, and I look at jit.gl.multiple's requirements, and one of the first things I think is, OK, how do I stick specific data of my choosing into matrices? To me it's a basic requirement, but also less convenient than you'd hope.
> Did you try it?
Yes, but it doesn't solve the data format problem.
hjh
OK... after evaluating the available options, I decided that probably the best way to go is:
1- create an abstraction to provide n-dimensional coordinates for setcell (rows before columns, e.g. 0 0, 1 0, 2 0, ... 10 0, 0 1, 1 1 etc.). I thought of a neat trick with [table] to do this without recursion, and without adding more objects for more dimensions.
2- then it's pretty straightforward to zl.iter a list of interleaved plane data, attach coordinates from this abstraction, "setcell" and done.
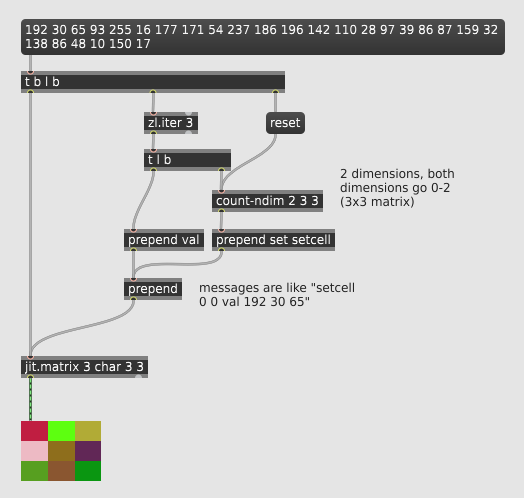
And that count-ndim.maxpat abstraction:
I think that's in pretty good shape, actually -- was looking for a way to make it not a pain in the hindquarters when I have to do it in class. The prepend stuff will bend the students' minds, but it isn't too much.
hjh
Hi James. If you use jit.coerce and jit.scanwrap you can solve most of the problems you're facing. Additionally, I think that the list to matrix conversion with jit.fill should have better performance than setting each cell individually.
Summary:
jit.fill stores the list in a 1-plane 1-dimension x cells matrix
jit.coerce interprets it as a 3-plane x/3 cells matrix
jit.scanwrap lets you wrap the 1-dimension matrix in x-dimensions (usually 2)
> If you use jit.coerce and jit.scanwrap you can solve most of the problems you're facing.
Oh wow, that's definitely a better solution. Letting the objects run the loops internally will definitely be faster.
This needs to be recorded in a "tips & tricks" list somewhere.
Thanks!
hjh
PS I'm also going to repost the count-ndim abstraction because I made a mistake with the size of the count-limits array. Fixed: