Switch Case Statement for input matching
Hey,
I'm still getting acquainted with creating Logic through signal flow in Max. I have the following scenario:
I am using a patcher with three different inlets. Depending on what the logical combination of these three inlets is, I'd like to send out a different message to the outlet of the patcher. What is least congested way of achieving this? I've been using a wild combination of "gate", "select" and "&&" objects but I always end up forgetting a scenario and I feel like having a completely separate signal path for each scenario is inefficient.
Is there a better way?
maybe matrix~ .
Looks cool, will test it out!
Thanks!
I actually ended up solving this by using javascript, since that was easier for me to wrap my head around. It works as intended now. However, just curious if someone would be up to look at the logic in my js object and let me know how I could have potentially achieved this with Max devices. Would appreciate that :))
// Define the number of inlets and outlets
inlets = 4; // 4 inlets: play, selector, range, loop
outlets = 4; // 4 outlets: 3 floats, 1 string
// Variables to store input values
var play = 0;
var selector = 0;
var range = 0;
var loop = 0;
var green = "Success Text";
var gray = "Device Background";
var red = "Automation";
var yellow = "Control On";
// Function to handle input
function msg_int(val) {
// Determine which inlet received the value
switch (inlet) {
case 0:
play = val;
break;
case 1:
selector = val;
break;
case 2:
range = val;
break;
case 3:
loop = val;
break;
}
// Process inputs and send outputs
processInputs();
}
// Function to process inputs and generate outputs
function processInputs() {
var output1, output2, output3, output4;
// If play is 0, default to these values
if (play == 0) {
output1 = 1.0;
output2 = 0.0;
output3 = 0.0;
output4 = gray;
} else {
// Check selector value
switch (selector) {
case 0: // NONE
output1 = 1.0;
output2 = 0.0;
output3 = 0.0;
output4 = green;
break; // Break to prevent fall-through
case 1: // STOP
output1 = 0.0;
output2 = 0.0;
output3 = 1.0;
output4 = red;
break;
case 2: // LOOP
if (range == 0) {
output1 = 1.0;
output2 = 0.0;
output3 = 0.0;
output4 = green;
} else {
if (loop == 1) {
output1 = 0.0;
output2 = 1.0;
output3 = 0.0;
output4 = yellow;
} else {
output1 = 0.0;
output2 = 1.0;
output3 = 0.0;
output4 = gray;
}
}
break;
default:
// Fallback in case the selector has an unexpected value
output1 = 0.0;
output2 = 0.0;
output3 = 0.0;
output4 = gray;
break;
}
}
// Send outputs
outlet(0, output1); // First float output
outlet(1, output2); // Second float output
outlet(2, output3); // Third float output
outlet(3, output4); // String output (color description)
}
I would do something like this:
Basically all inputs are first stored into a [i], and then the cascade is triggered by checking the value of each input with [sel], starting by play
.
It's worth noticing that play 1 && selector 0
gives the same 1 0 0 green
output as play 1 && selector 2 && range 0
.
if the inputs are bools (0 or 1) and the output should be a single int for each possible combination, that would be indentical to a binary to decimal number conversion.
however, for only 3 "digits" it should be fine to hardcode that using logic/select/i.
or what about a compromise between these two worlds? :)
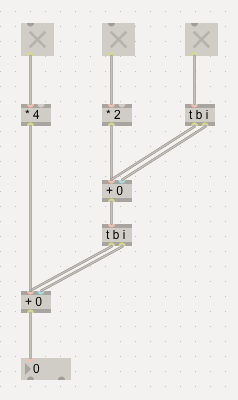
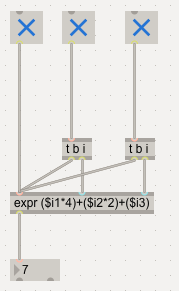
input 2 has range 0 - 2.
can't make a 4bit binary out of it ...
I would simply stuff coll with messages,
and use requested combinations of that 4
inputs to recall them.