Generate an array of pairs
Hii tried to generate a number of arrays based on msg_int.Not sure what im doing tbh need some advice.
I think my prop1 prop2 could be breaking something
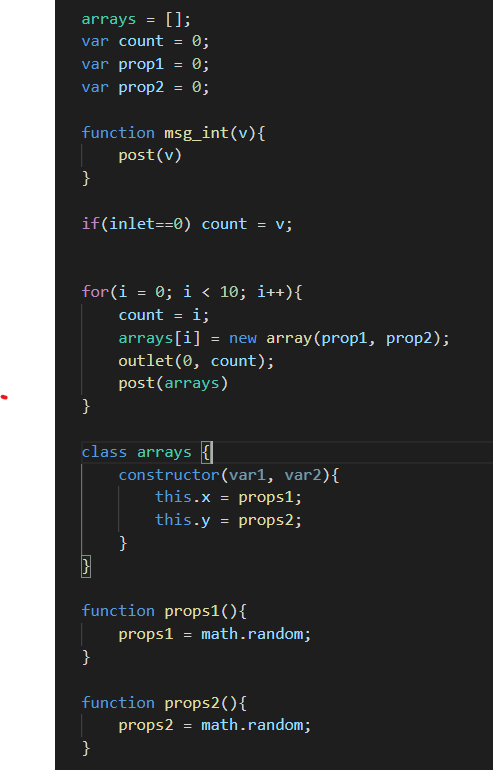
A few quick thoughts:
1. To my knowledge, Max does not support Javascript Class statement
2. Javascript is case sensitive. "new array" should be "new Array"
3. To call a function you must follow the function name with an argument list enclosed in parens "()" (even if the list is 0 in length.
4. If you expect a function to return a value, you must use the return statement.
5. To generate a random number it's Math.random(), not math.random.
You also have some issues around the scope of your variables. I'm guessing you want your if statement and your for loop inside the msg_int function (or within their own function and called from the msg_int function.
Good luck!
"To my knowledge, Max does not support Javascript Class statement" - I found the same, but you can define a class using the function statement like so (this is the protoype-creation method mentioned below):
function myPair()
{
this.prop1 = 0.0;
this.prop2 = 0.0;
}
then you build an Array by instantiating these objects (again, you might also push into the Array, as shown below, but I would push objects, not just values):
var pairArray = new Array();
for(var i = 0; i < arraySize; i++)
{
pairArray[i] = new myPair(); // this is where the object is created
pairArray[i].prop1 = 0.0;
pairArray[i].prop2 = 0.0;
}
then use the Array like so:
var myID;
function setID(n)
{
myID = n;
}
function prop1(n)
{
pairArray[myID].prop1 = n;
}
function prop2(n)
{
pairArray[myID].prop2 = n;
}
Why not just do this?
var randomNumberPair = [];
// then while the array is empty, execute this for loop
for (i=0; i<10; i++);
{
randomNumberPair.push([Math.random(), Math.random()]);
outlet (0, i);
post(randomNumberPair[i]);
}
But regarding classes, JavaScript in Max does support objects built with prototypes, and I think that might help. Prototypes take the places of classes in JavaScript, since you can build objects with constructors and what not.
OP was asking about structs/objects with named properties, so the object-creation with member properties is definitely the route to go.
https://www.w3schools.com/js/js_object_prototypes.asp