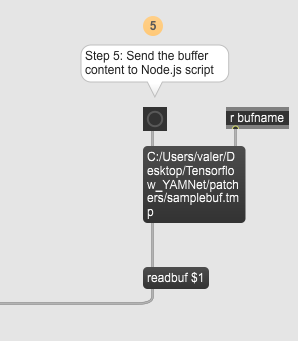
Try to change manually the content of the message in /Users/walidbreidi/Documents/Max 8/XxPatches_and_ResourcesxX/Valerio Orlandini/Tensorflow_YAMNet/patchers/samplebuf.tmp. Since it features spaces, you may need to enclose the path in double quotes.